Merging PDF files with Python´s pypdf
This tiny Python program merges multiple PDF files within a directory into a single PDF file to make life easier.
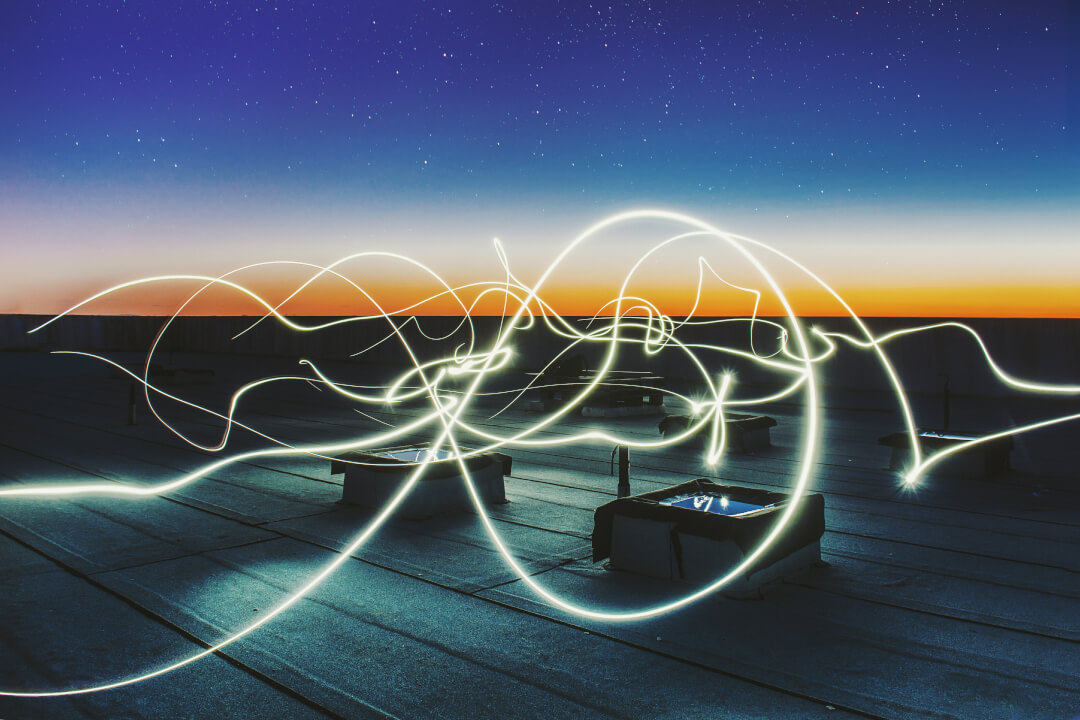
If you are travel and want to print something on paper in the copyshop, you can put your PDF files on a USB memory stick and print from it in the store.
If you have only a few documents, this is usually not a problem, but if you have a lot of them you might want to have everything in a single PDF document. Then it's quite simply enough to open this document and print it out only once.
Github Repository of the Python Code
All said and done, what´s the point of being a programmer? With Python and the very helpful PDF library pypdf you can write a quick and dirty application. With pypdf you can not only create PDF documents, but also merge already existing PDF documents.
The following Python program creates a list of all existing PDF files in a folder and creates a new single PDF with all connected documents in a sub-directory.
from os import mkdir
from os.path import join
from pathlib import Path
from pypdf import PdfMerger
path = './test'
pdf_merger_path = Path(join(path, 'merged'))
filename = 'Merged_PDF_File.pdf'
try:
filelist = sorted([f.name for f in Path(path).iterdir() if f.is_file() and f.suffix.lower() == '.pdf'])
if len(filelist) > 0:
if filename[-4:] == '.pdf':
pdf_merger = PdfMerger()
for count, value in enumerate(filelist):
pdf_merger.append(join(path, value))
print(f"{count + 1}. {value} merged.")
if not pdf_merger_path.is_dir():
mkdir(pdf_merger_path)
pdf_merger.write(join(pdf_merger_path, filename))
print(f"==> Merged {count + 1} PDF file{'' if count == 0 else 's'}: {filename} created inside {pdf_merger_path} directory.")
else:
print(f"Incorrect filename {filename} for PDF files.")
else:
print(f"No PDF files(s) found in the {path} directory.")
except FileNotFoundError:
print (f"Directory {path} not found.")
The small program can be easily customized to your own needs, for example, automatically adding a watermark or the number of pages.